Reimagine Digital Experience & Commerce
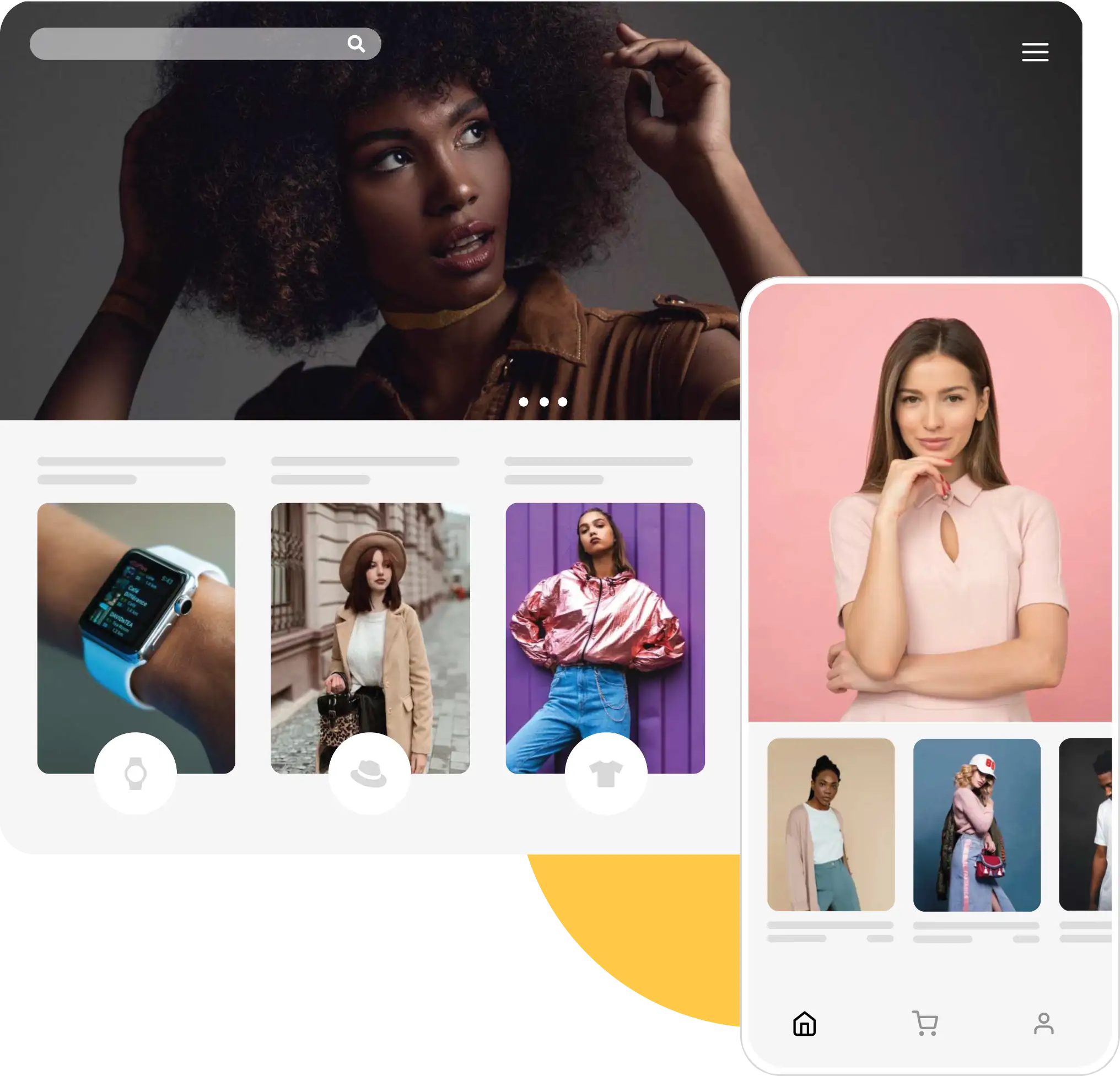
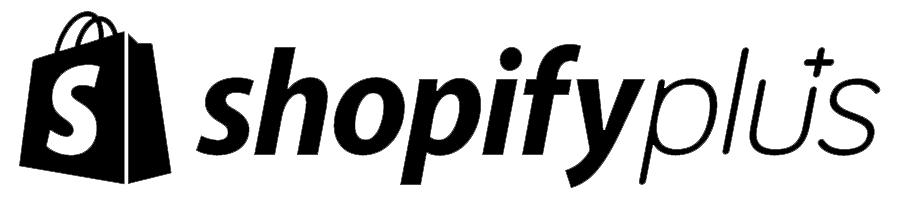
about
Challenging status-quo and debunking mediocrity in ecommerce technology since inception
We saw technology challenges hampering the growth of ecommerce initiatives of many successful organizations. Most of these organizations were engaging technology partners who either sold pre-packaged solutions without considering the business goals or those who built e-commerce solutions just like any other piece of software.
At Codilar, we believe building technology for ecommerce is a totally different ball game. A partner needs to have sheer focus and deep understanding of the nuances in customer experience, performance, conversion, data analytics, SEO etc in addition to strong technical expertise to build enterprise-grade features and integrations.
We envisaged a truly focused digital commerce agency that specializes in the world’s best ecommerce platform Magento (Adobe Commerce) while equipping ourselves with deep knowledge of all the factors that influence the performance and success of ecommerce initiatives.
A quick glimpse at some of the best outcomes which elevated the brands
We are the go to agency for organizations to get started or grow in their digital commerce presence. We guide you through the journey at all stages.
We inculcated the best practices backed up with years of experience in the domain to deliver unparalleled solutions. Here is a quick glimpse of some of the work done for our awesome clients.
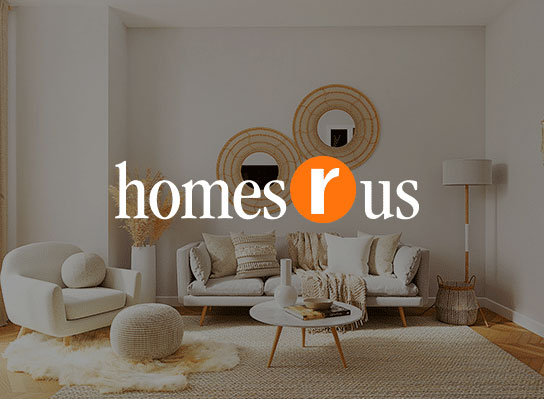
Homes r Us
Multi-country ecommerce implementation for Middle East’s fastest growing home furniture brand on Adobe Commerce and Pimcore Explore project →
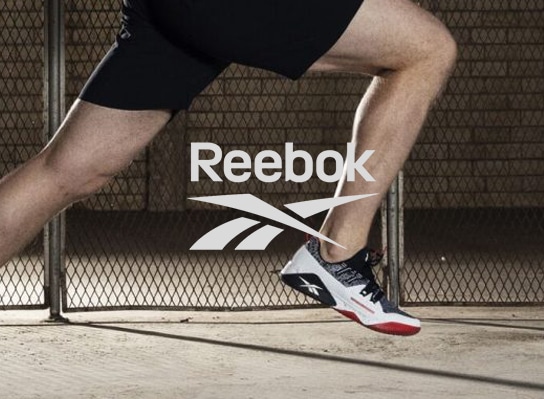
Reebok
Reebok, a global athletic footwear and apparel company, wanted to create a new ecommerce website to sell their products online. They approached us to build the website on Magento Cloud and customize it to meet their specific needs. Explore project →
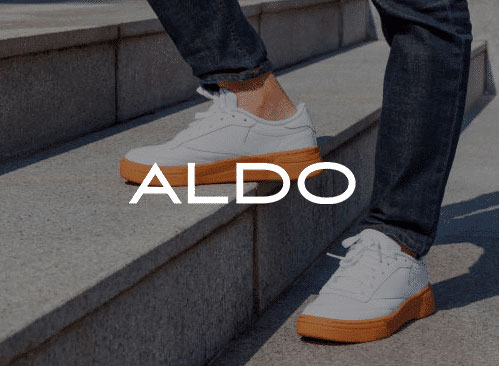
Aldo
Omnichannel ecommerce implementation for world’s favourite footwear & accessories brand in 4 countries Explore project →
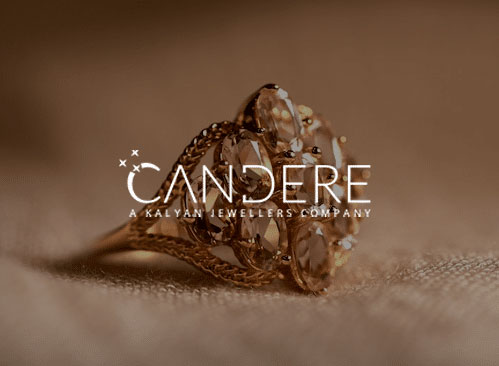
Candere
Enterprise grade ecommerce solution for the largest brand on Adobe Commerce. Explore project →
We focus on users and their experience first
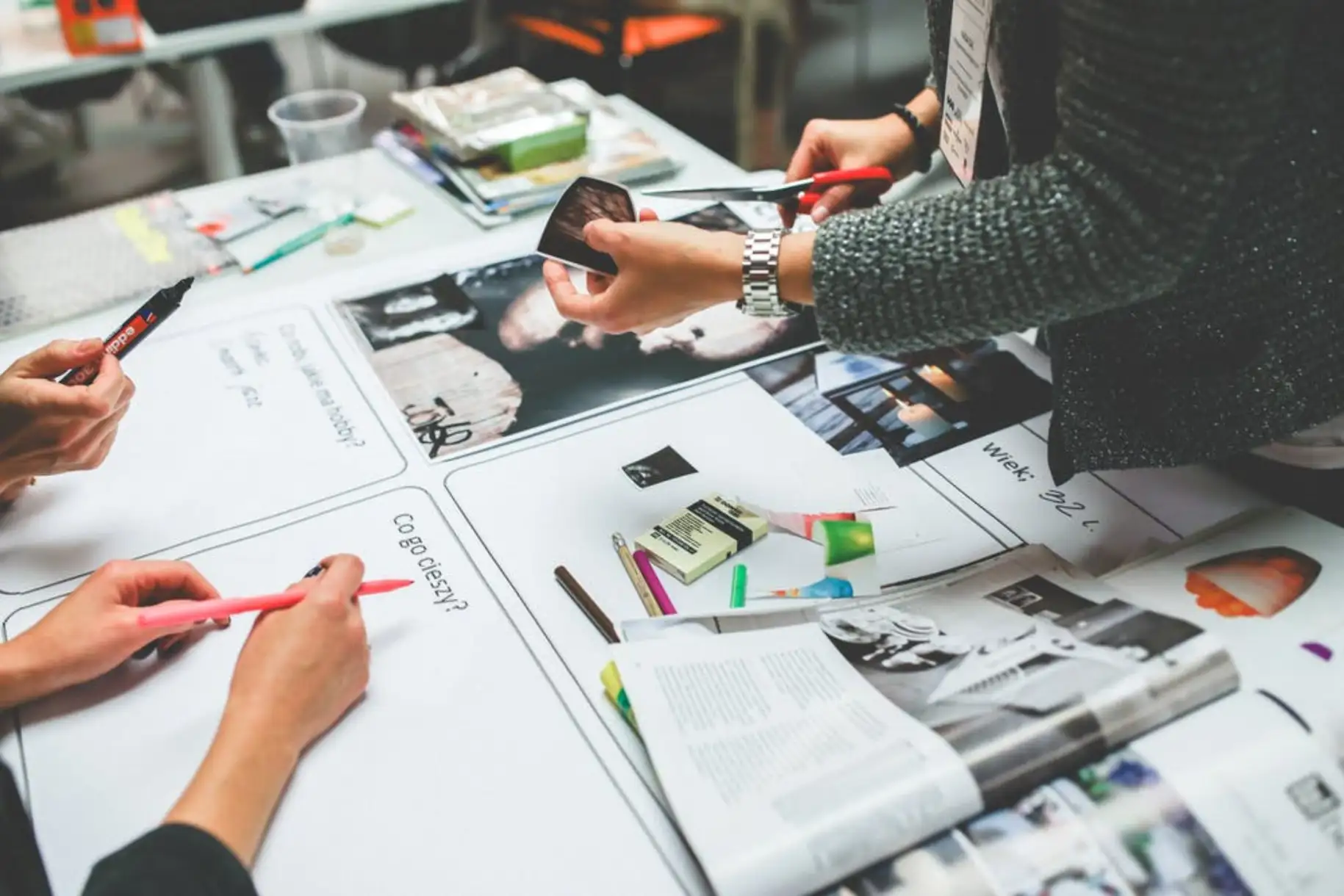
Empathy
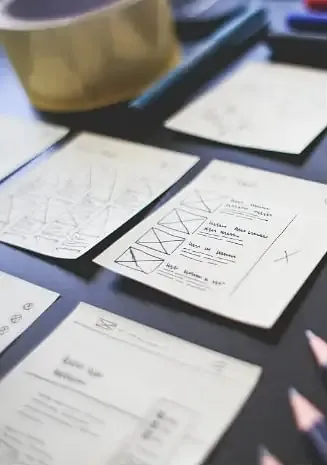
UX Design
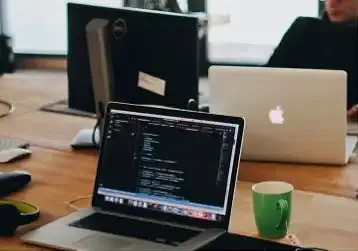
Pixel, Code and Business
Though we advocate users, we also believe it the business decisions and the code implications. We adapt and deliver the best experience
1. Platforms
- Adobe Commerce (Magento)
- Shopify Plus
- Pimcore
- Akinon
- Adobe Experience Cloud
2. Digital Commerce
- Headless Architecture
- Composable Commerce
- B2C & Omnichannel
- D2C
- B2B Commerce
- Mobile Commerce
- Order Management System (OMS)
3. Service
- Strategy Consulting
- Experience Design
- Platform Engineering
- Digital Marketing
- DevOps
- Conversion Rate Optimization
- Managed Support
4. Content & Data
- Digital Experience (DXP)
- Analytics
- Product Information Management (PIM)
- Customer Data Platform (CDP)
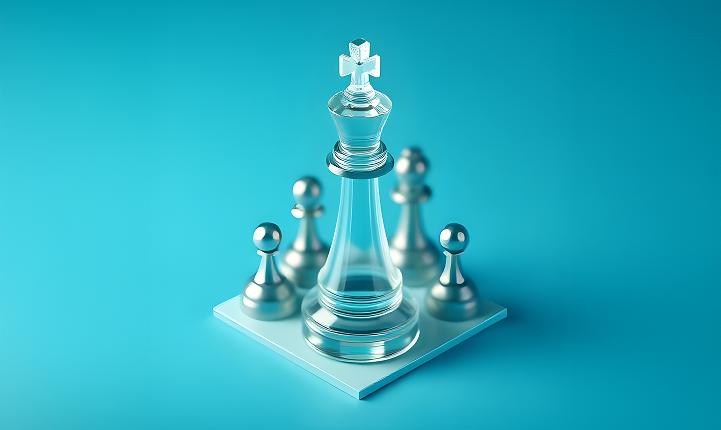
why Codilar
Pioneering performance commerce
Performance Commerce is a concept invented by us that drives our delivery strategy. We define it as “a development culture that keeps business success as the key focus”.
We believe that technology solutions for digital commerce have to be developed very differently compared to other software. There are several factors such as user experience, speed, analytics, SEO, content, security, consumer psychology and conversions that influence the success and performance of an ecommerce platform.
At Codilar, we strive to thoroughly understand the “success” for each of our clients. We train daily to strengthen our skills and ensure the designs and code we craft for our clients contribute to this success.
Sheer Focus
Enterprise-Grade
Building highly scalable and reliable stores with complex modules and integrations is our forte.
Holistic Approach
World-class code, data, performance and consumer psychology are at the heart of our craft.
Agile
Real Success in the Real World
Our unique and proven execution methodology has enabled the delivery of a customized eCommerce solution.
Testimonials
See what our customers are saying
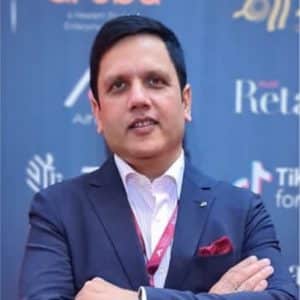
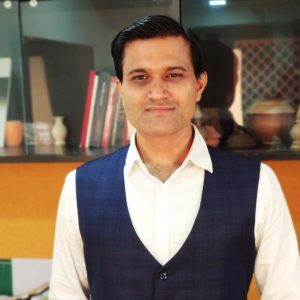
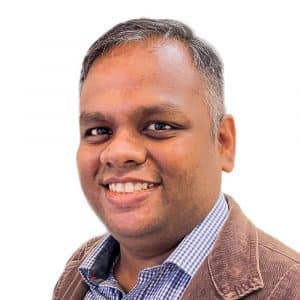
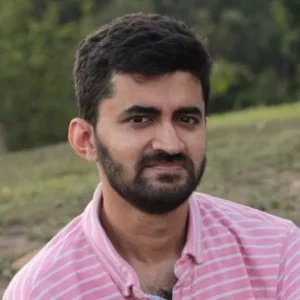
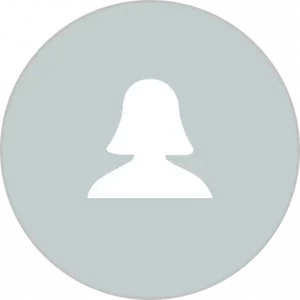
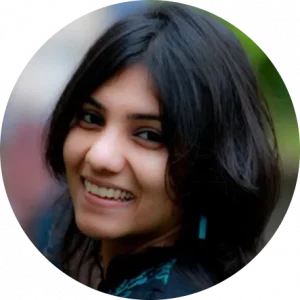
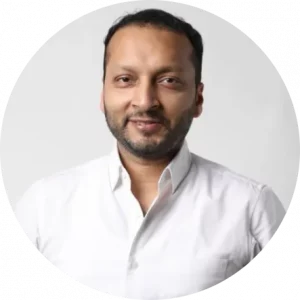
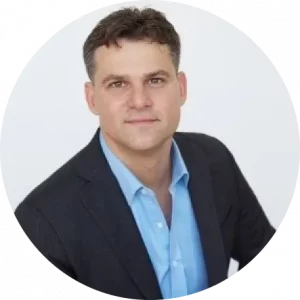
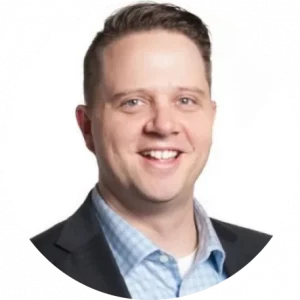
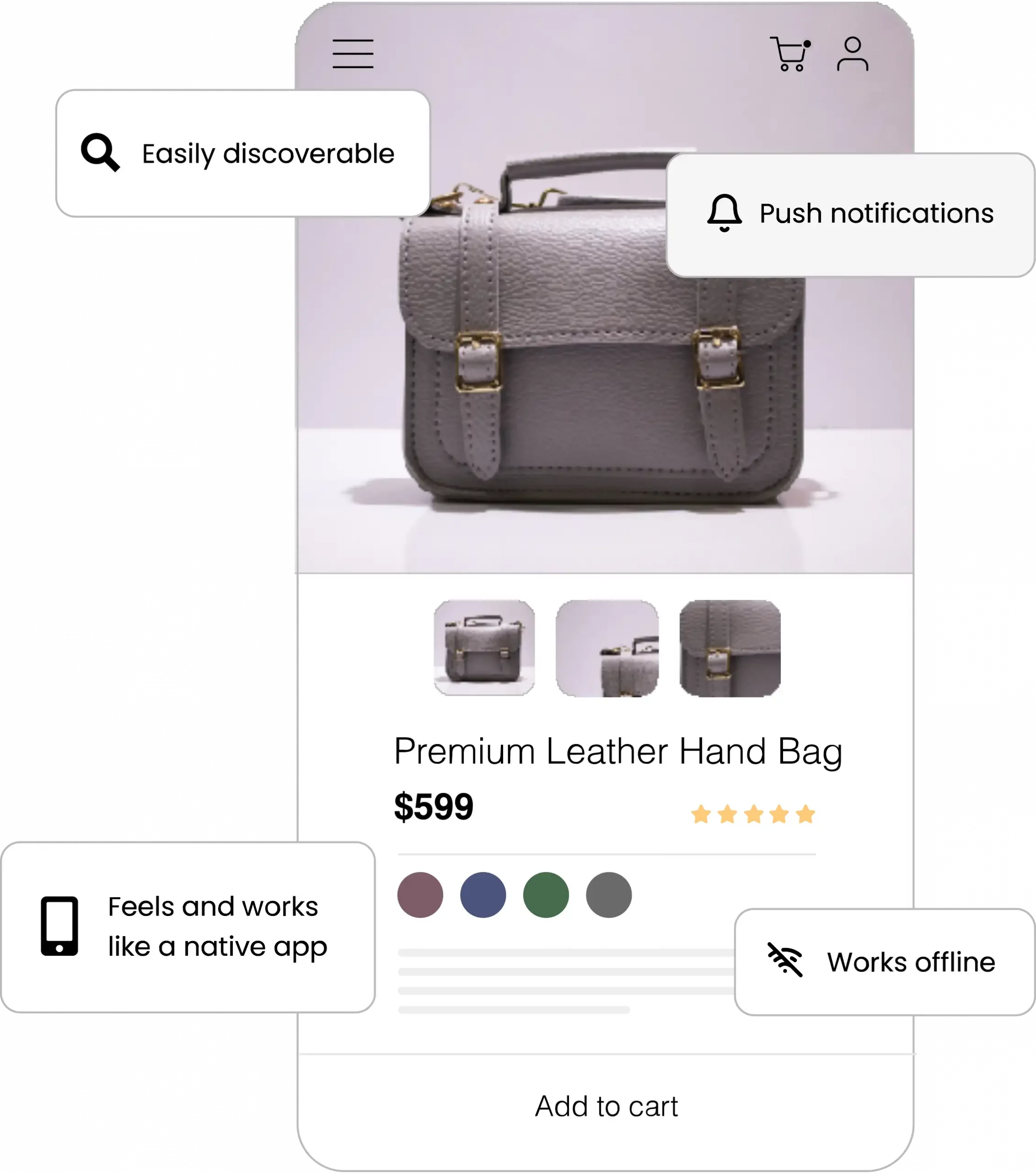
Introducing NexPwa A superfast PWA for Magento to boost conversions
- Built for Magento
- Low Investment. Faster Go-To-Market One-Time Purchase
- Implementation, Training & Support
- No Additional Infrastructure
- Google Pagespeed Score 90+ and built in SEO
- Payment Integration
- RTL Support
Stories
Stay up to date with the latest eCommerce & Magento updates and more...
You’re in good company
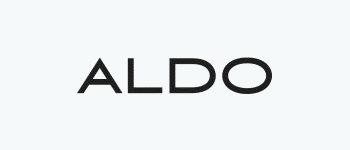
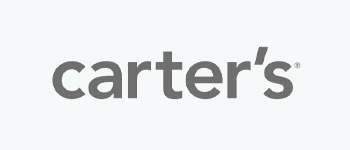
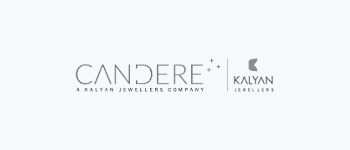
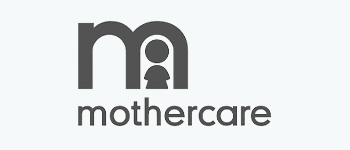
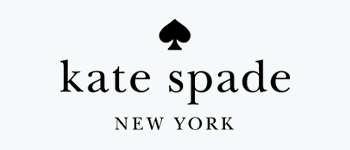
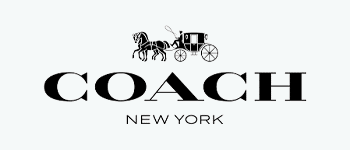
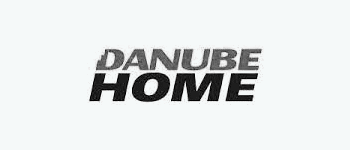
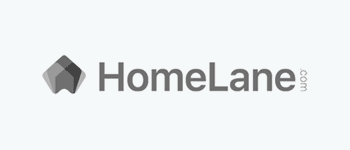
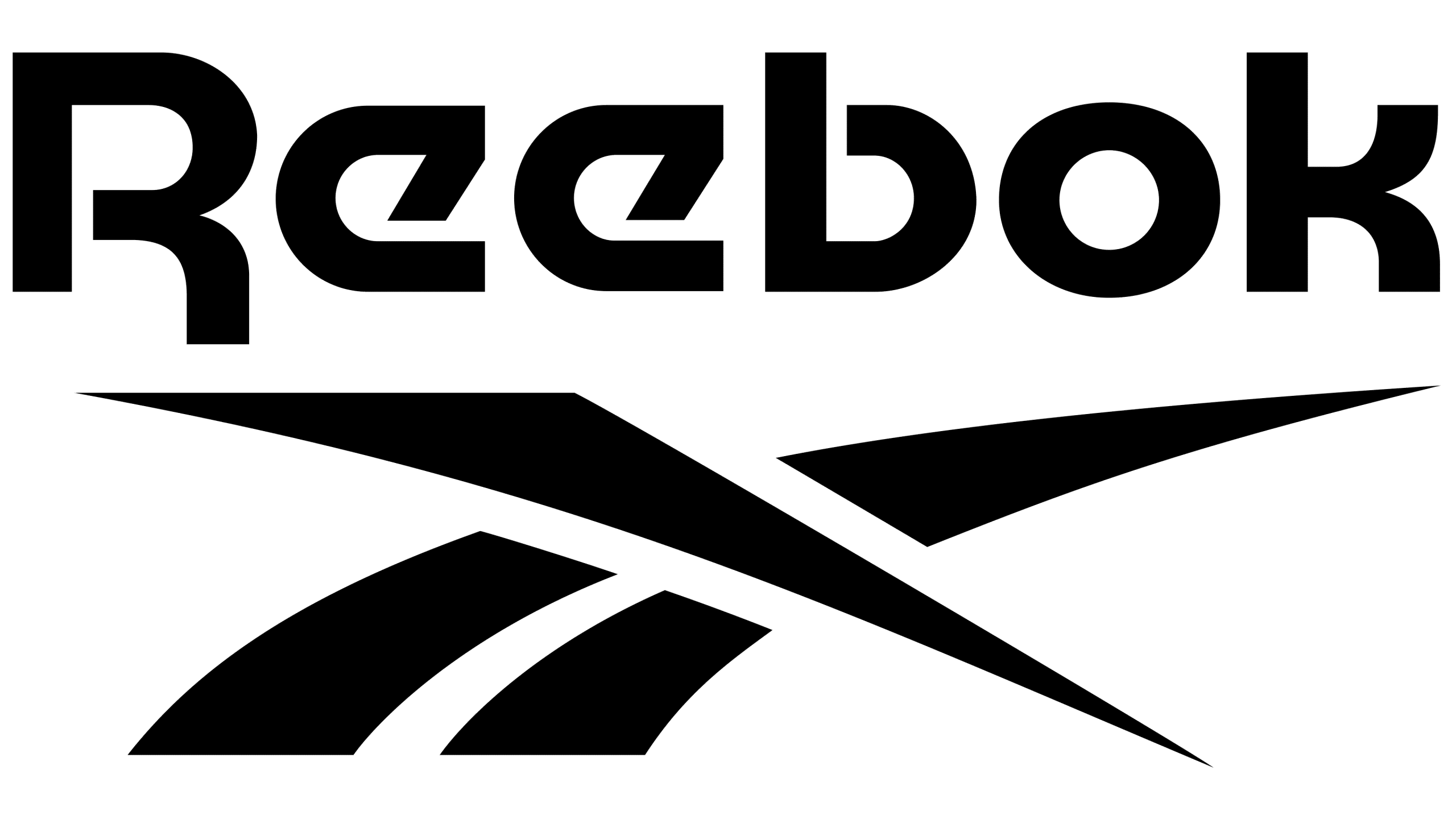
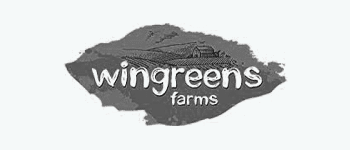
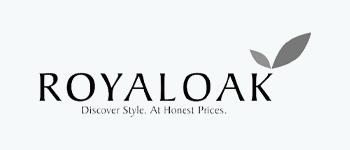
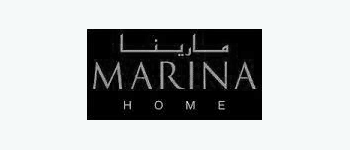
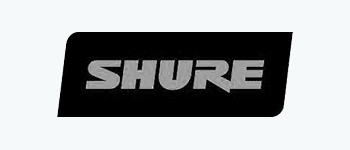
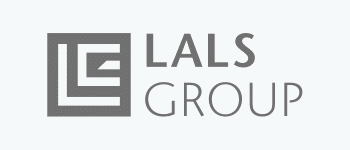
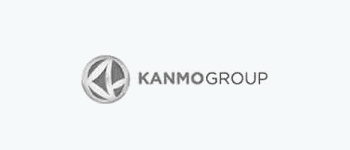
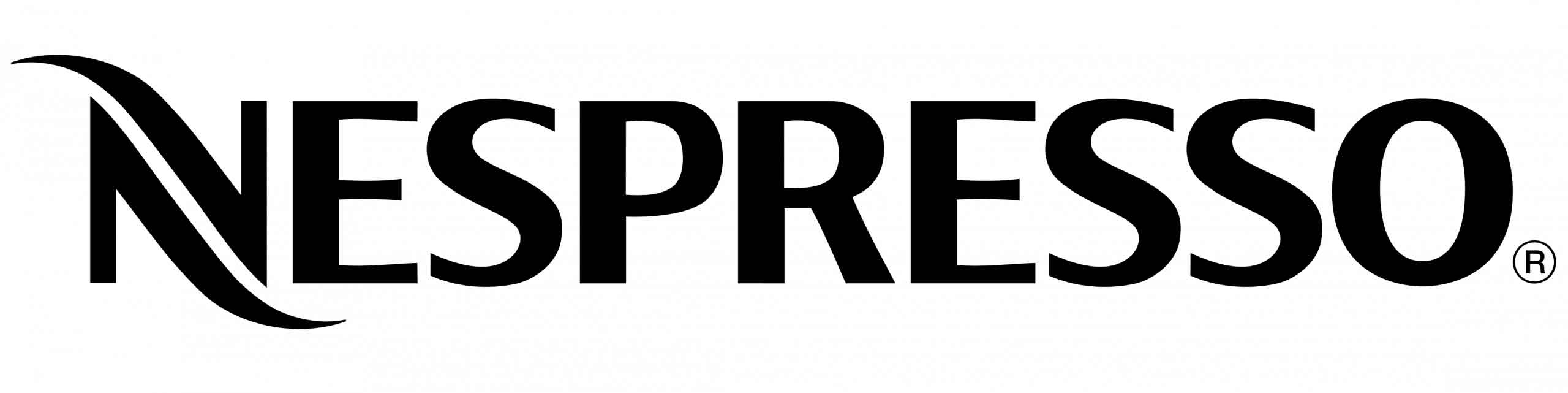
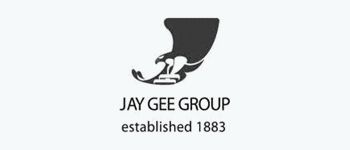
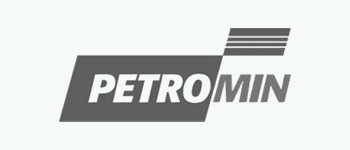
Keep growing. We’ve got your back
Award winning team
We are not just another team with coding skills. Our team comprises the best talent which bagged us awards.
100+ ecomm solutions
Our expertise in the domain and the verticals have resulted in delivering diverse solutions across the globe.
Squad of Magento ninjas
We call ourselves ninjas just because we feel like it. Yes, the vast powerful experience and skills keep us a cut above.